mirror of
https://github.com/FluuxIO/go-xmpp.git
synced 2024-11-21 10:02:00 -08:00
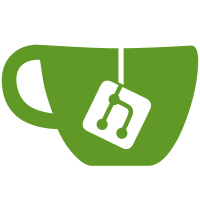
"An IQ stanza of type "get" or "set" MUST contain exactly one child element, which specifies the semantics of the particular request."
130 lines
3.3 KiB
Go
130 lines
3.3 KiB
Go
package xmpp_test
|
|
|
|
import (
|
|
"encoding/xml"
|
|
"strings"
|
|
"testing"
|
|
|
|
"github.com/google/go-cmp/cmp"
|
|
"gosrc.io/xmpp"
|
|
)
|
|
|
|
func TestUnmarshalIqs(t *testing.T) {
|
|
//var cs1 = new(iot.ControlSet)
|
|
var tests = []struct {
|
|
iqString string
|
|
parsedIQ xmpp.IQ
|
|
}{
|
|
{"<iq id=\"1\" type=\"set\" to=\"test@localhost\"/>",
|
|
xmpp.IQ{XMLName: xml.Name{Space: "", Local: "iq"}, PacketAttrs: xmpp.PacketAttrs{To: "test@localhost", Type: "set", Id: "1"}}},
|
|
//{"<iq xmlns=\"jabber:client\" id=\"2\" type=\"set\" to=\"test@localhost\" from=\"server\"><set xmlns=\"urn:xmpp:iot:control\"/></iq>", IQ{XMLName: xml.Name{Space: "jabber:client", Local: "iq"}, PacketAttrs: PacketAttrs{To: "test@localhost", From: "server", Type: "set", Id: "2"}, Payload: cs1}},
|
|
}
|
|
|
|
for _, test := range tests {
|
|
parsedIQ := xmpp.IQ{}
|
|
err := xml.Unmarshal([]byte(test.iqString), &parsedIQ)
|
|
if err != nil {
|
|
t.Errorf("Unmarshal(%s) returned error", test.iqString)
|
|
}
|
|
|
|
if !xmlEqual(parsedIQ, test.parsedIQ) {
|
|
t.Errorf("non matching items\n%s", cmp.Diff(parsedIQ, test.parsedIQ))
|
|
}
|
|
|
|
}
|
|
}
|
|
|
|
func TestGenerateIq(t *testing.T) {
|
|
iq := xmpp.NewIQ("result", "admin@localhost", "test@localhost", "1", "en")
|
|
payload := xmpp.DiscoInfo{
|
|
Identity: xmpp.Identity{
|
|
Name: "Test Gateway",
|
|
Category: "gateway",
|
|
Type: "mqtt",
|
|
},
|
|
Features: []xmpp.Feature{
|
|
{Var: xmpp.NSDiscoInfo},
|
|
{Var: xmpp.NSDiscoItems},
|
|
},
|
|
}
|
|
iq.Payload = &payload
|
|
|
|
data, err := xml.Marshal(iq)
|
|
if err != nil {
|
|
t.Errorf("cannot marshal xml structure")
|
|
}
|
|
|
|
if strings.Contains(string(data), "<error ") {
|
|
t.Error("empty error should not be serialized")
|
|
}
|
|
|
|
parsedIQ := xmpp.IQ{}
|
|
if err = xml.Unmarshal(data, &parsedIQ); err != nil {
|
|
t.Errorf("Unmarshal(%s) returned error", data)
|
|
}
|
|
|
|
if !xmlEqual(parsedIQ.Payload, iq.Payload) {
|
|
t.Errorf("non matching items\n%s", cmp.Diff(parsedIQ.Payload, iq.Payload))
|
|
}
|
|
}
|
|
|
|
func TestErrorTag(t *testing.T) {
|
|
xError := xmpp.Err{
|
|
XMLName: xml.Name{Local: "error"},
|
|
Code: 503,
|
|
Type: "cancel",
|
|
Reason: "service-unavailable",
|
|
Text: "User session not found",
|
|
}
|
|
|
|
data, err := xml.Marshal(xError)
|
|
if err != nil {
|
|
t.Errorf("cannot marshal xml structure: %s", err)
|
|
}
|
|
|
|
parsedError := xmpp.Err{}
|
|
if err = xml.Unmarshal(data, &parsedError); err != nil {
|
|
t.Errorf("Unmarshal(%s) returned error", data)
|
|
}
|
|
|
|
if !xmlEqual(parsedError, xError) {
|
|
t.Errorf("non matching items\n%s", cmp.Diff(parsedError, xError))
|
|
}
|
|
}
|
|
|
|
func TestDiscoItems(t *testing.T) {
|
|
iq := xmpp.NewIQ("get", "romeo@montague.net/orchard", "catalog.shakespeare.lit", "items3", "en")
|
|
payload := xmpp.DiscoItems{
|
|
Node: "music",
|
|
}
|
|
iq.Payload = &payload
|
|
|
|
data, err := xml.Marshal(iq)
|
|
if err != nil {
|
|
t.Errorf("cannot marshal xml structure")
|
|
}
|
|
|
|
parsedIQ := xmpp.IQ{}
|
|
if err = xml.Unmarshal(data, &parsedIQ); err != nil {
|
|
t.Errorf("Unmarshal(%s) returned error", data)
|
|
}
|
|
|
|
if !xmlEqual(parsedIQ.Payload, iq.Payload) {
|
|
t.Errorf("non matching items\n%s", cmp.Diff(parsedIQ.Payload, iq.Payload))
|
|
}
|
|
}
|
|
|
|
func TestUnmarshalPayload(t *testing.T) {
|
|
query := "<iq to='service.localhost' type='get' id='1'><query xmlns='jabber:iq:version'/></iq>"
|
|
|
|
parsedIQ := xmpp.IQ{}
|
|
err := xml.Unmarshal([]byte(query), &parsedIQ)
|
|
if err != nil {
|
|
t.Errorf("Unmarshal(%s) returned error", query)
|
|
}
|
|
|
|
if parsedIQ.Payload == nil {
|
|
t.Error("Missing payload")
|
|
}
|
|
}
|